When writing software, code documentation is practically required.
These documents are essentially a cheat sheet for your software, helping render the complex architecture of the code into more digestible concepts.
With code documentation, anyone can read your codebase and understand its structure, dependencies, and purpose.
The texts are a medium for knowledge sharing and help with code management.
However, the code documentation needs to be well-written for your developers to reap these benefits. Subpar documents aren’t of use to anyone.
That’s what this article is for: we’ll walk you through some tips on writing stellar code documentation that developers will love.
Write Clean Code First
The purpose of code documentation is to explain code so that it’s easily understood and more manageable.
However, code is complex, and it’s challenging to write digestible documentation about it. The practice is often a specialized skill, and many hire technical writers for this specific task and for better technical documentation.
Now imagine if the code lacked consistency in the naming, syntax conventions, and functions. Such messy code would make writing documentation that much harder.
Therefore, to achieve high-quality code documentation, you first need high-quality code. Clean code is the prerequisite for code documentation.
A good starting point is the architecture of the codebase, as a logical folder structure allows developers to navigate the codebase easily.
Here’s a sample of React's folder organization:

There are several intuitive main folders, such as assets, components, pages, etc. Each parent folder is then broken down into child folders, which all relate to the parent folder.
For example, the components folder contains folders grouped by elements like buttons, custom input fields, and similar.
Likewise, the parts folder is divided into reusable snippets (footers, headers, etc.).
With this clean, coherent organization, developers can quickly and efficiently navigate the codebase and locate the code they need.
As to the actual code itself, the following acronym stands for some good advice:
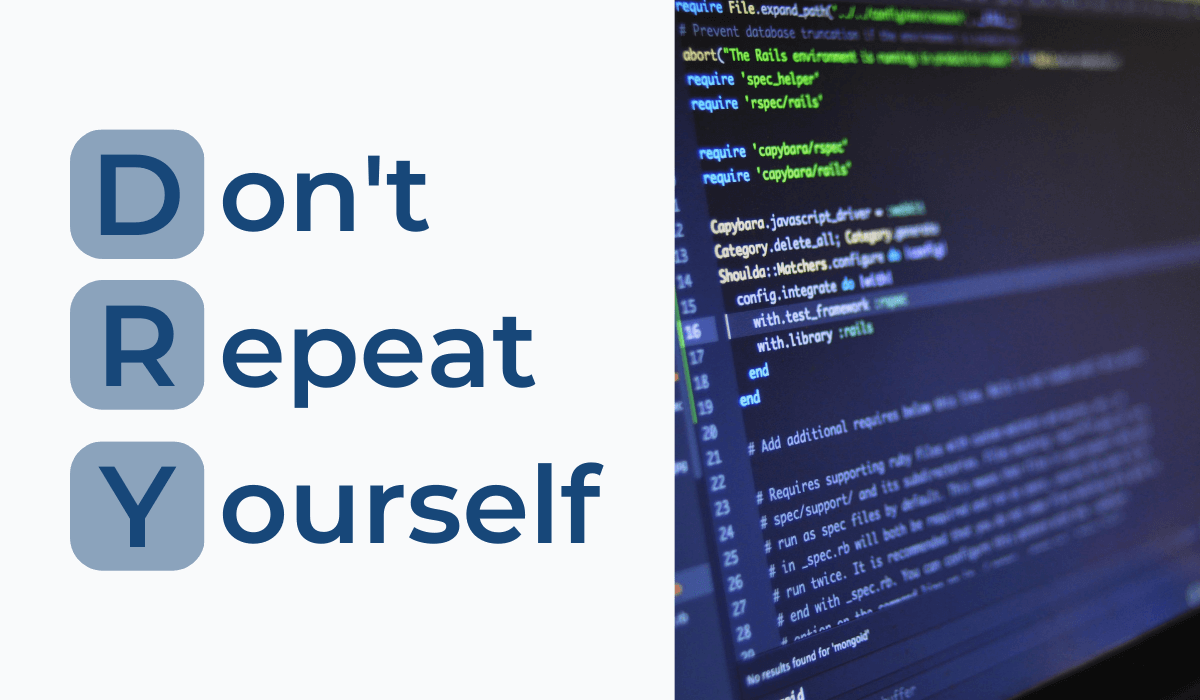
DRY—don’t repeat yourself—advocates that no two lines of code should be identical.
This is because multiple lines of duplicate code automatically increase the codebase, therefore making it difficult to maintain.
Imagine your developer needs to edit non-DRY code.
Instead of changing a single line, they would have to systematically adjust every line of code in a net of endless repeated, duplicated code.
Kasun Koswatta offers a better alternative:

Instead of constantly repeating functionalities, a more elegant solution is to create a method or class that can enact that functionality multiple times.
That way, the codebase is smaller and more manageable yet accomplishes the same action.
While writing your DRY code, it’s also essential to focus on variable names. Variables should be named descriptively, so there’s no ambiguity regarding the code’s purpose.
Short, simple names such as data or customer can mean almost anything and will likely confuse developers.
Instead, it’s better to employ variable names such as:

These detailed names indicate the code’s exact functionality, and most developers will know what the variable is referring to.
With these clear-cut, clean variable names, the code should be easy to read and understand, and even easier to document.
Add Code Comments
Even if your codebase is the cleanest it could possibly be, there will still be times when it’s not immediately apparent what the code does or why it does it.
Your developers will occasionally have to deviate from standard coding practices for a multitude of reasons, and these instances will look odd to fresh eyes despite there likely being adequate justification.
This is where code comments are helpful: they’re the perfect space to explain the logic behind code. Comments help developers to understand why the code was written the way it was.
Such documentation is critical, as it will help your peers understand your code better. Author Hal Abelson illustrated why this is so essential:
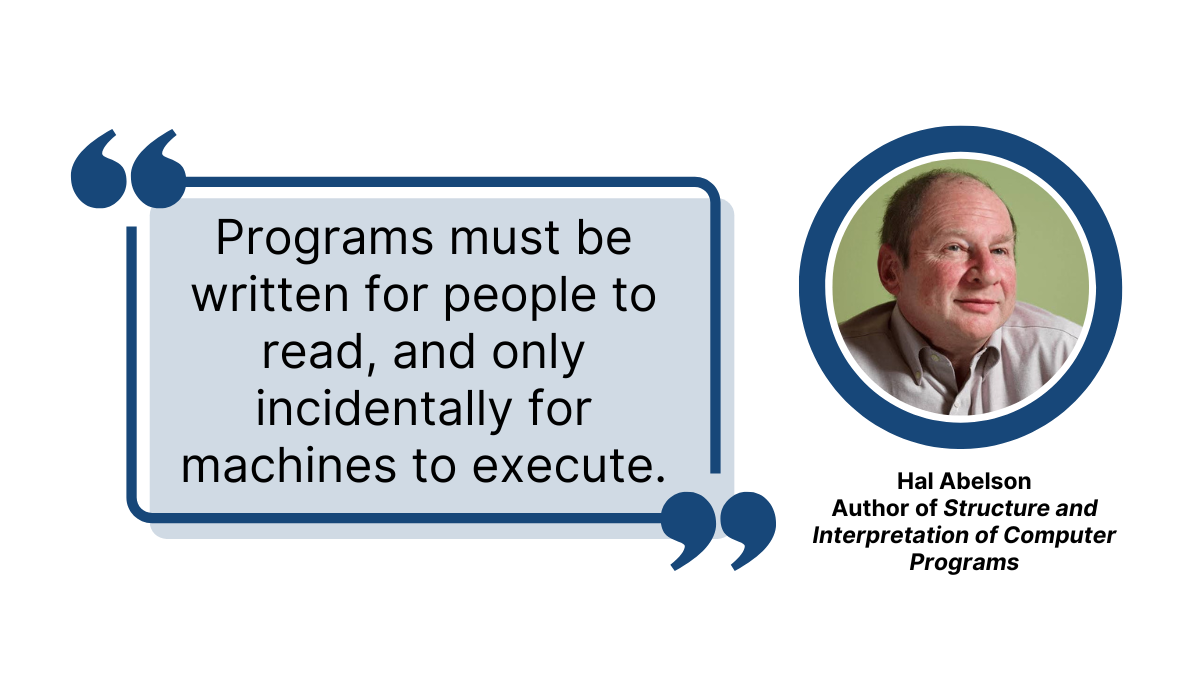
The hallmark of high-functioning software is that humans easily understand it; otherwise, it’s not a very sophisticated program. Here's why you need to write very good software documentation!
Comments are a fantastic medium for enabling this understanding.
The following snippet proves how effective comments can be:

This tidbit of information is a niche, surprising revelation. Most developers would have automatically reverted to the global function simply because it’s the most common practice.
However, because of this helpful comment, all newcomers to the codebase will immediately know why the Number.isFinite function was used.
Furthermore, developers would have likely lost significant time without this note, stalling further development.
Hours would have been spent discovering this peculiarity, as opposed to the five seconds it takes to read this comment.
If you’re unsure how to begin writing such code comments, there are several tools to help you out.
For example, GhostDoc is a Visual Studio extension that automatically generates XML comments from the source code.
In other words, your developers won’t have to annotate the code themselves. Instead, one keystroke will do the trick.
Here’s how it works:
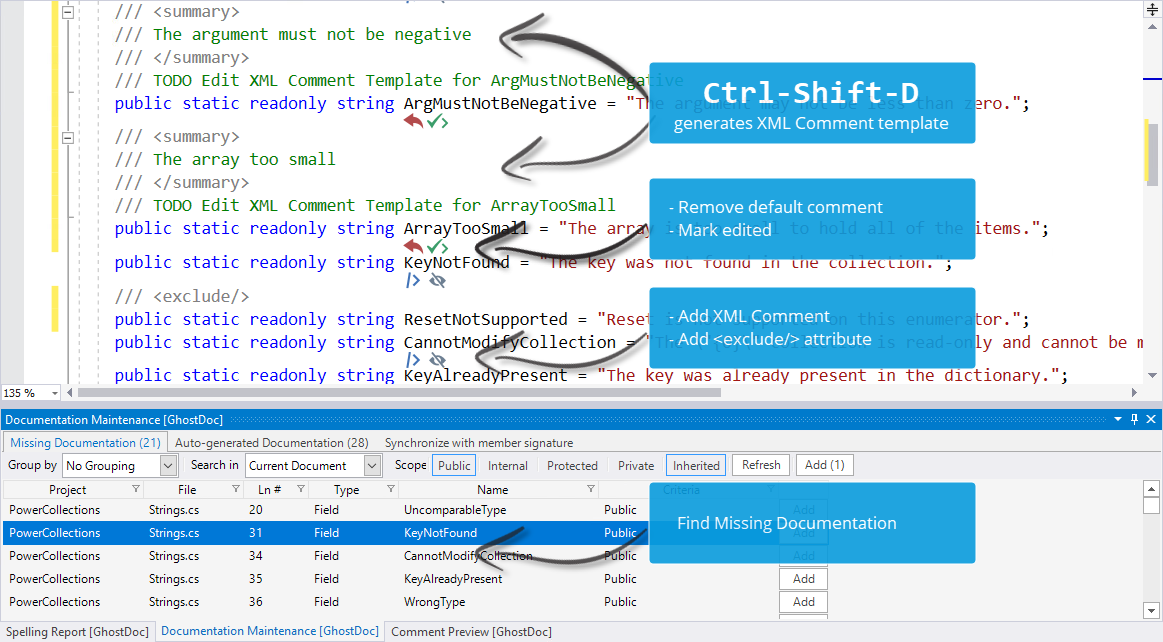
The tool highlights missing documentation, removes default comments (there’ll be an icon indicating this), and, most importantly, generates XML comment templates.
With this resource, your developers will have extensively-commented code in no time, creating an accessible, transparent codebase anyone can understand and contribute to.
Choose the Right Documentation Tool
Strange as it may seem, you’d have difficulties writing code documentation using Microsoft Word.
This is because code documentation requires advanced features often lacking in standard word processing tools. Consequently, it’s worth investing in a specialized code documentation tools.
For example, Archbee is a documentation platform that hosts all your company’s documents while providing features to facilitate the writing process.
One such feature is a multi-language code editor: a block containing multiple tabs to display code snippets across various languages.
Here’s an example:
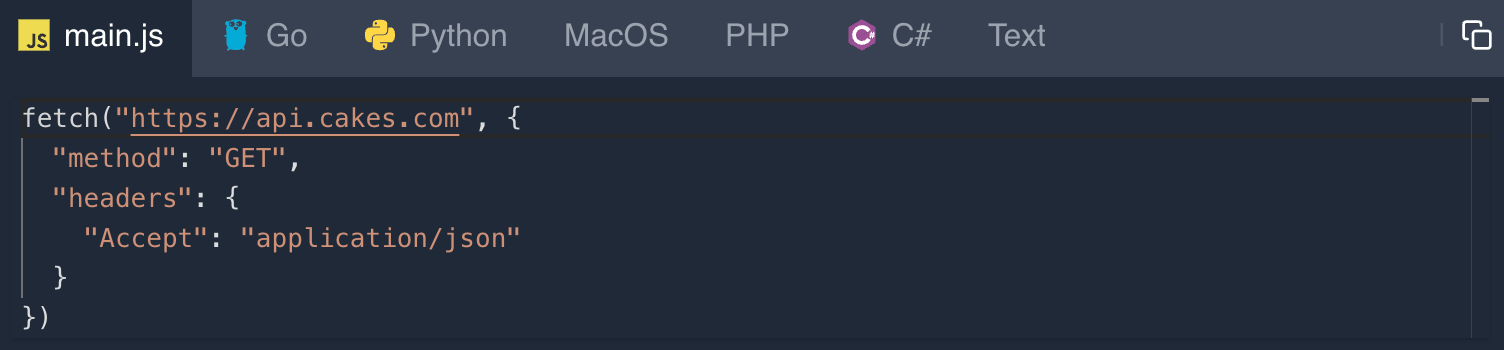
This example showcases JavaScript, Go, C#, and more, all in a single code block.
Archbee also offers Code Drawers, a popular interactive block layout. With this feature, code samples change as you scroll down the page, and the code drawer enters the viewport.
Take a look:

This feature is ideal for lengthier documents with multiple code examples, as it allows for a clearer overview.
Archbee is an ideal solution for manually documenting code. However, if you’re short on time, some tools will automatically generate documentation for you.
For instance, Dozygen independently creates documentation from the source code, extracting information from the comments added to the code.
Since the documentation is derived from the annotations, it’s easy to maintain consistency with the source code.
Look at this sample code:

This is its Doxygen-generated documentation:
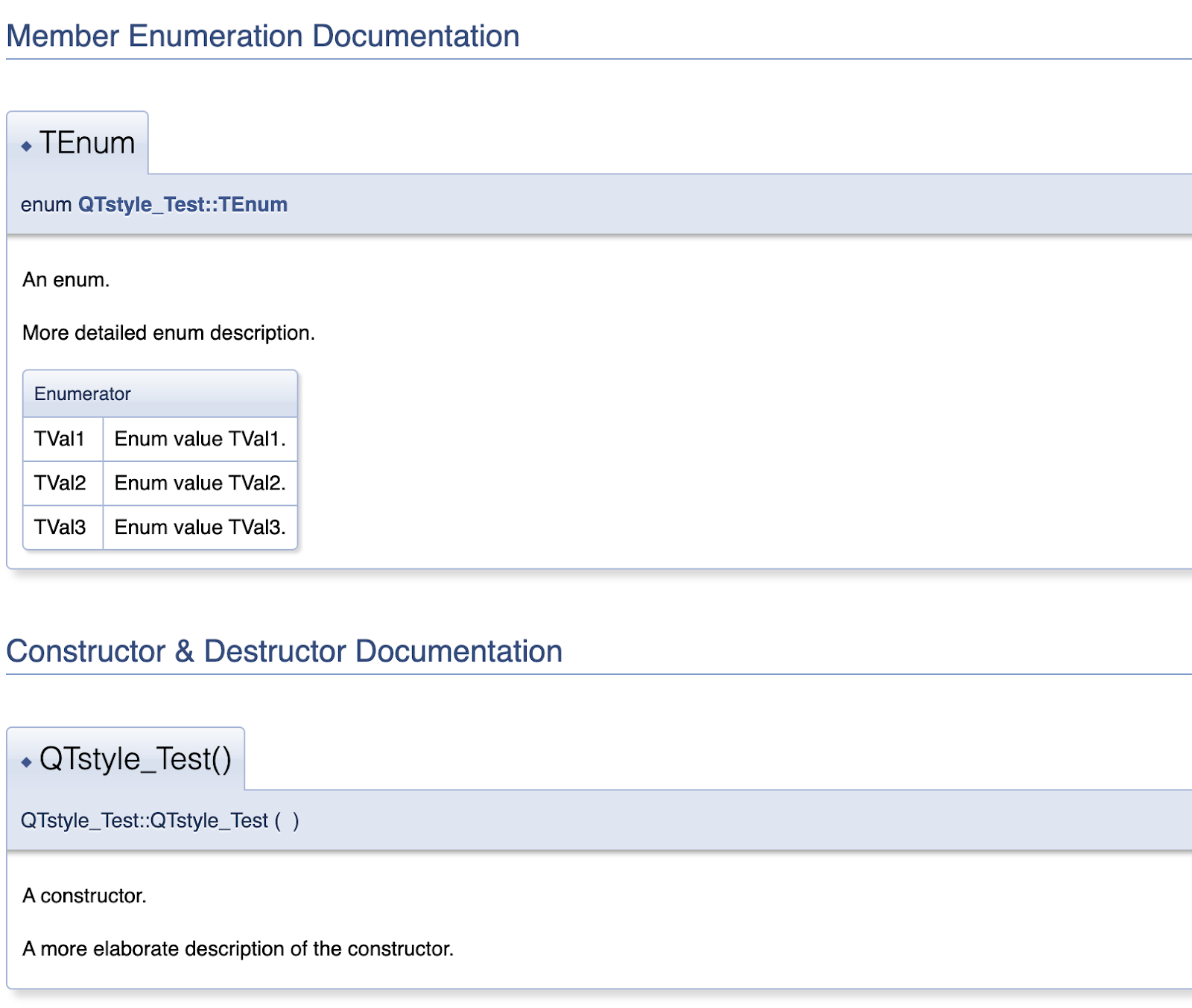
Doxygen can create documentation in an online browser (in HTML) or an offline reference manual (in LaTeX). The above image depicts the online browser option.
This documentation tool is handy when you don’t have much time, as the documents are automatically created without manual work.
Document as You Write
Code is always the most intelligible while you’re writing it. With complex functions, even revisiting it after a few days' break can result in head-scratching as you struggle to decipher your logic from earlier in the week.
In other words, the rationale and reasoning behind your code are most evident in the moment of composition.
As a result, it’s a good idea to document as you code simply because that’s when the information is clearest to you.
Victoria Drake, the Principal Software Engineer at Sophos, also commented on this:
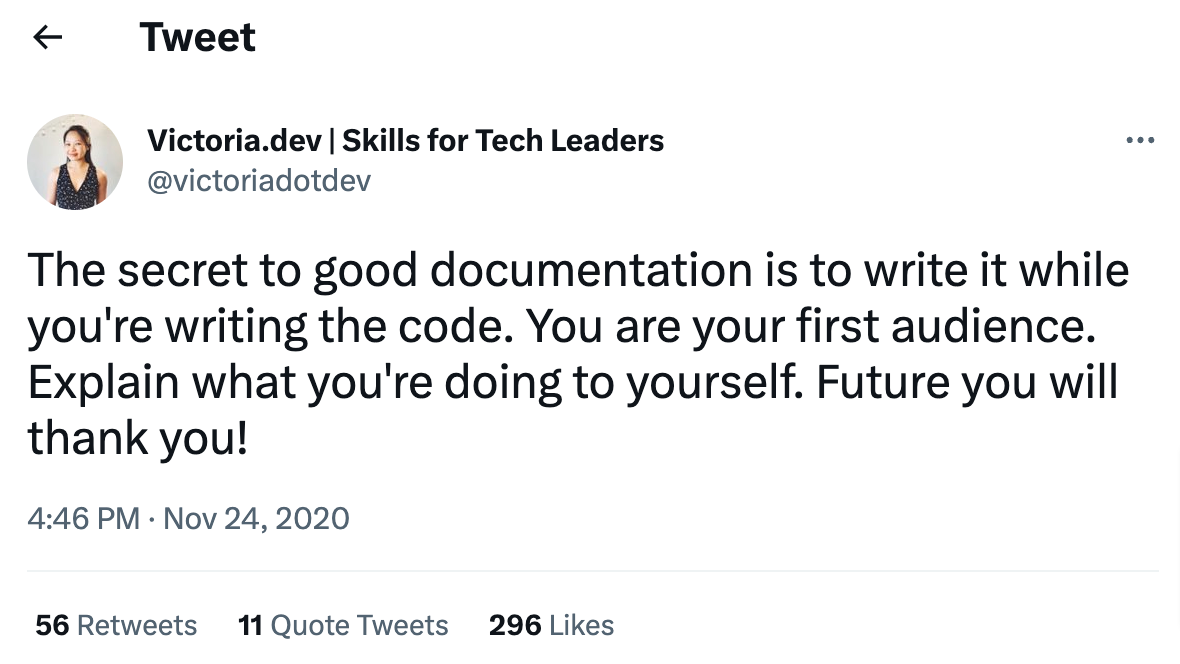
You’ll always be the most knowledgeable about the code when writing the code. As time goes on, your memory will degrade more and more.
As such, it’s vital to utilize this advantage and record your insights when they’re clearest.
In a Quora discussion on this subject, analyst Jim Brinsley echoed this sentiment, stating:
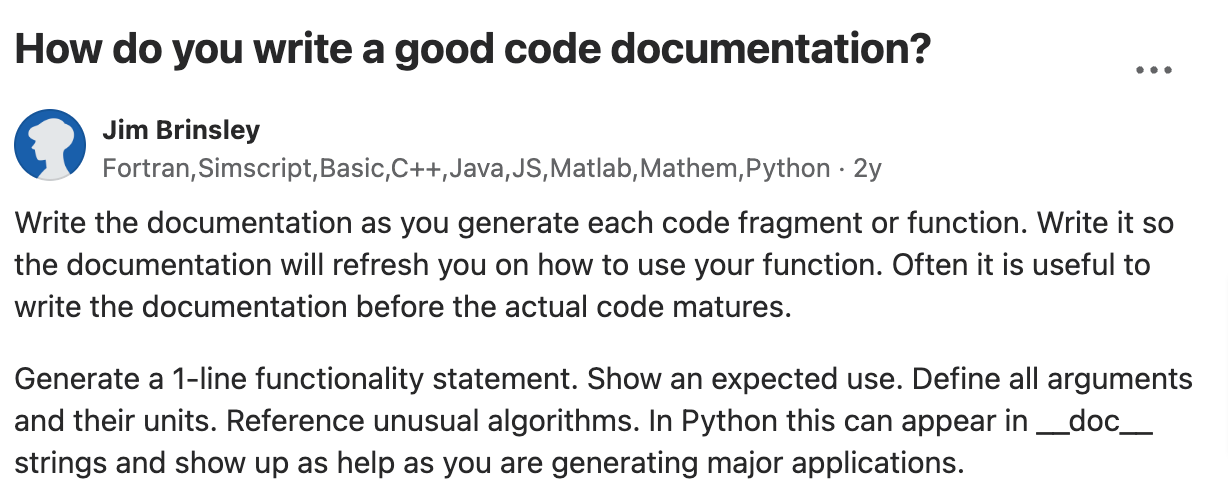
Documentation should ideally be written after each code fragment or function to ensure a comprehensive, detailed guide on the entire codebase.
Examples of helpful code documentation best written immediately are unusual algorithms, argument definitions, and expected uses.
This data can be forgotten later on but should be fresh in your mind while initially writing the code.
This topic was also discussed in a Reddit post, where a user highlighted the dangers of documenting at the wrong time:

If you document before writing the code, you’ll likely have to backtrack and edit the documentation upon finishing coding.
After all, you never know what unexpected alterations you’ll have to make due to changed requirements or unforeseen roadblocks.
However, if you document after writing the code, you’ll again have to revisit the documentation once more after debugging or implementing new functionalities.
Furthermore, as previously mentioned, the information won’t be as fresh as when writing the code.
In any case, you’ll likely be reworking the documentation in both scenarios.
Documenting while coding is the only approach that ensures you won’t constantly be revisiting and editing the documentation.
Focus on the Ease of Understanding
Code documentation should be detailed and clear enough for anyone to understand the code it describes.
Even new employees or external developers should grasp your software’s architecture, regardless of their individual skills and experience.
Therefore, code documentation’s chief characteristic should be intelligibility.
Ideally, the texts would assume the reader knows nothing about what the code does, why it does it, and how it works. Consequently, everything should be written in plain language.
Or, as Tim Peters put it:

The main goal of documentation is to explain complex concepts in clear, digestible language. However, that’s not always possible, as software is complicated by nature. Here's what makes writing code documentation a challenge and get ready!
In such situations, it’s crucial to manage the complexity effectively; to present the complexities without complicating them further.
If you’re unsure how to do so, here’s one effective method:
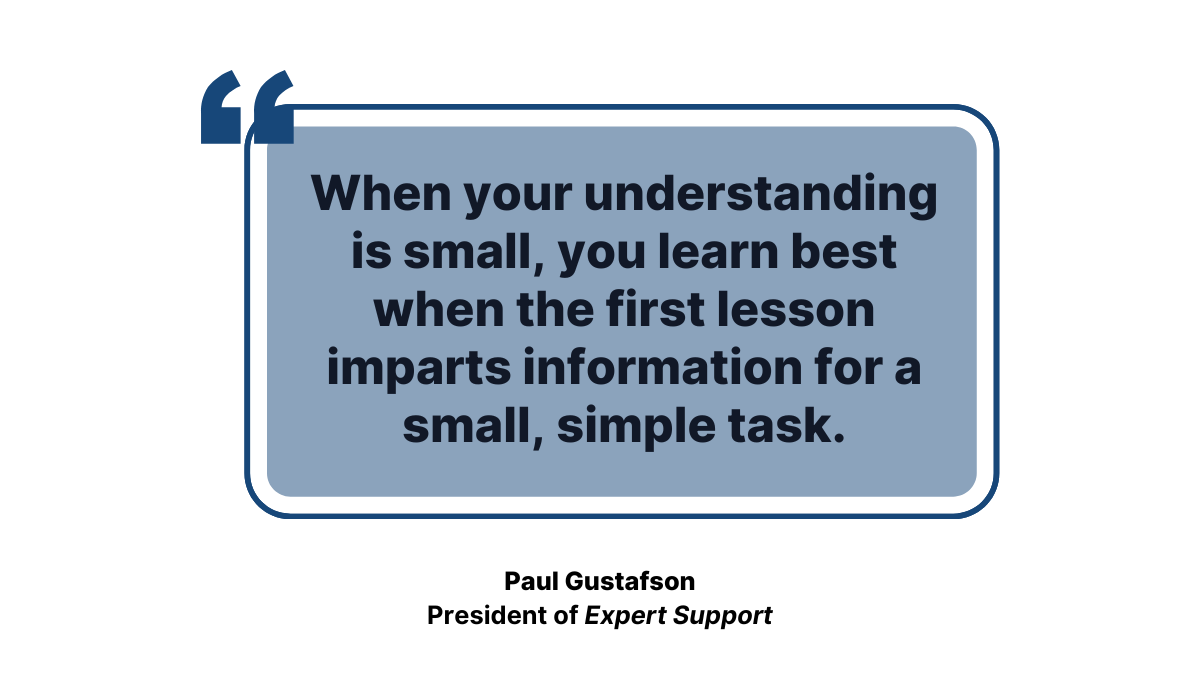
The key is to begin the documentation with small, bite-sized explanations that are easily understood.
Then, work your way through more complex concepts, adding additional information each time.
With this incremental strategy, you avoid overwhelming the reader with too much information at once and slowly yet surely introduce them to the software’s inner workings.
Twilio does this well. The below image shows the first page of their REST APIs documentation:
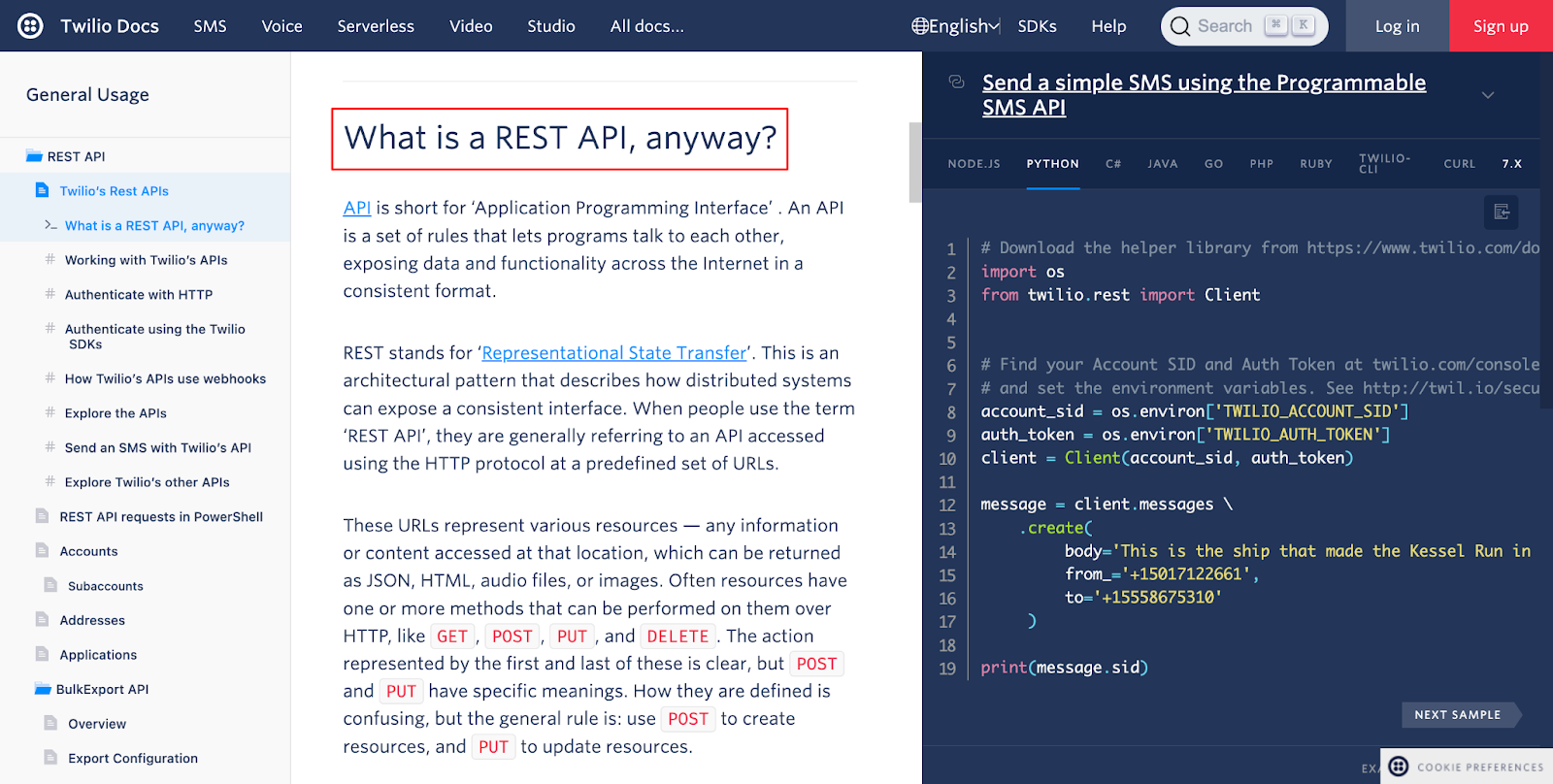
Before explaining how to use their APIs, Twilio takes a step back and first explains what an API is in the first place.
This might be an oversimplification for some developers. Still, for less-experienced readers, the clarification is a great introduction and will help them more easily understand the rest of the text.
Furthermore, Twilio incorporates code samples that automatically render as the user scrolls down the document (i.e., code drawers). Here’s an example:
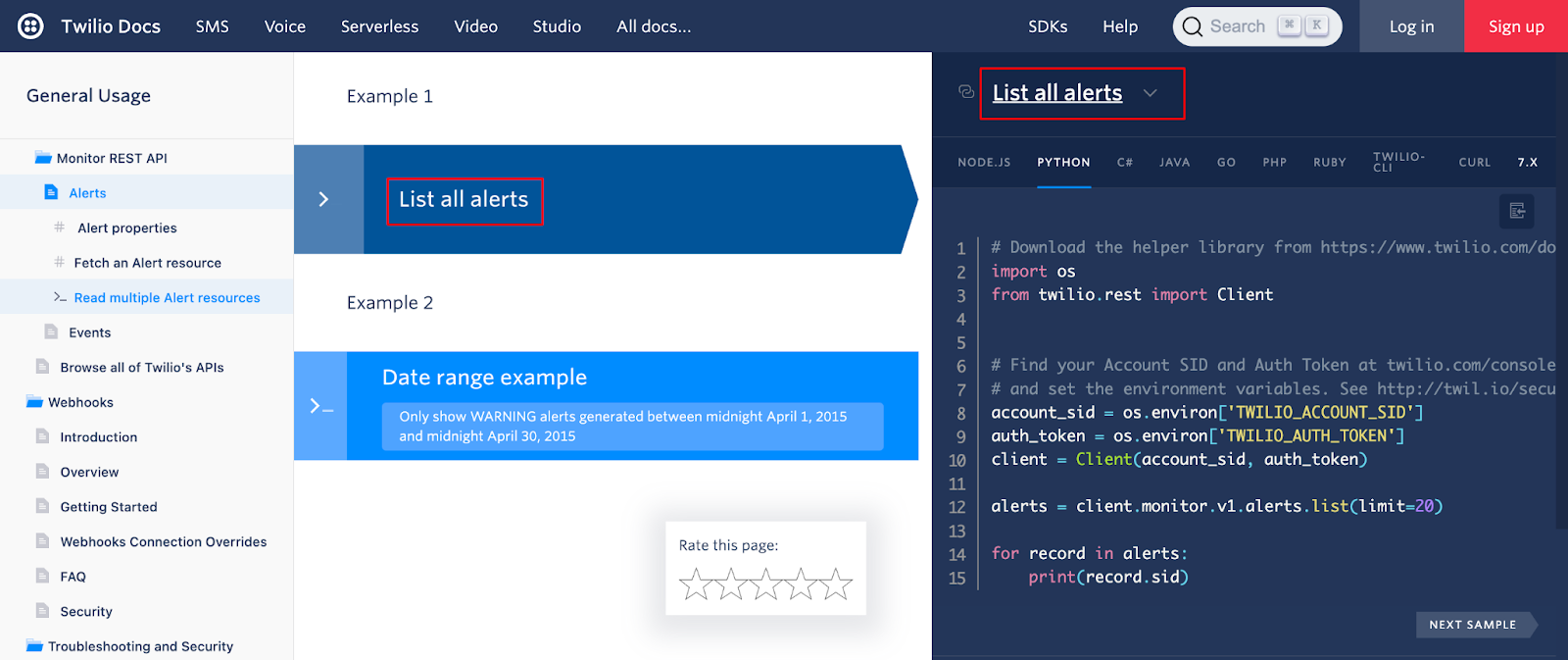
This code sample reveals how to list all alerts, but a brief scroll will change the code into a snippet illustrating date range examples.
The interactive, clear layout allows readers to easily view code samples without abandoning the plain-text explanation.
The dual-screen increases readability and makes it much easier to understand the software’s capabilities.
Before we finish, here's a blog post about best practices in code documentation for software engineers.
Conclusion
Writing code documentation is a huge help when developing software.
These documents help translate intricate systems into more straightforward portions; they essentially walk developers through the software. With code documentation as a guiding light, developers can easily manage and edit the software they’re working in.
For example, code comments explaining codebase peculiarities can save hours of work. In addition, if the documentation is written simultaneously with coding, it’s guaranteed to cover every codebase detail.
Well-written code documentation is often the key to effective collaboration and software development. Make sure your employees document well, and you’re guaranteed to build high-functioning software.